Creating Custom EVM Scenarios
Learn how to create, share, and contribute custom EVM execution scenarios to help others understand complex blockchain interactions.
What are EVM Scenarios?
EVM Scenarios are structured representations of Ethereum transactions and their execution steps. They allow you to visualize how the EVM processes transactions, manipulates stack, memory, and storage, and updates the world state.
Scenarios are particularly useful for educational purposes, debugging complex transactions, and understanding how different smart contract interactions work under the hood.
Scenario Structure
An EVM Scenario consists of a transaction definition and a series of execution steps. Each step represents a point in time during the transaction execution, with specific values for stack, memory, storage, and other EVM components.
import type { EVMScenario } from "@/types/scenarios";
// Example scenario definition
const myScenario: EVMScenario = {
// Basic information
name: "Simple Value Transfer",
description: "Basic ETH transfer between two accounts",
// Transaction details
transaction: {
from: "0x742d35Cc6634C0532925a3b844Bc454e4438f44e",
to: "0x1234567890123456789012345678901234567890",
value: "0.1",
gasLimit: 21000,
gasPrice: 20,
data: "0x",
nonce: 5,
},
// Execution steps
steps: [
{
description: "Transaction initiated",
opcodes: [],
stack: [],
memory: "",
storage: {},
},
{
description: "Pushing value to stack",
opcodes: ["PUSH1 0x60"],
stack: ["0x60"],
memory: "",
storage: {},
},
{
description: "Storing value in memory",
opcodes: ["MSTORE"],
stack: [],
memory: "0x0000000000000000000000000000000000000000000000000000000000000060",
storage: {},
},
// More steps...
],
};
Scenario Fields
Each scenario consists of the following fields:
- name: A descriptive name for the scenario (e.g., 'ERC-20 Token Transfer')
- description: A detailed explanation of what the scenario demonstrates
- transaction: The transaction details that initiate the scenario
- from: The sender's Ethereum address
- to: The recipient's Ethereum address (or contract address)
- value: The amount of ETH to transfer (as a string)
- gasLimit: The maximum gas allowed for the transaction
- gasPrice: The gas price in Gwei
- data: The transaction data (bytecode or encoded function call)
- nonce: The transaction nonce
- steps: An array of execution steps, each containing:
- description: A description of what happens in this step
- opcodes: The EVM opcodes executed in this step
- stack: The state of the stack after this step
- memory: The state of memory after this step (as a hex string)
- storage: The state of storage after this step (as a key-value object)
- worldState (optional): Changes to the world state in this step
- gasUsed (optional): Gas used up to this step
- gasRemaining (optional): Gas remaining after this step
Creating Scenarios
There are two ways to create custom scenarios:
Using the Scenario Builder UI
The EVM Visualizer includes a built-in Scenario Builder that allows you to create scenarios through a user-friendly interface:
- Launch the EVM Visualizer
- Click the "Create Custom Scenario" button in the top toolbar
- Fill in the basic information and transaction details
- Add execution steps with their respective stack, memory, and storage states
- Preview and save your scenario
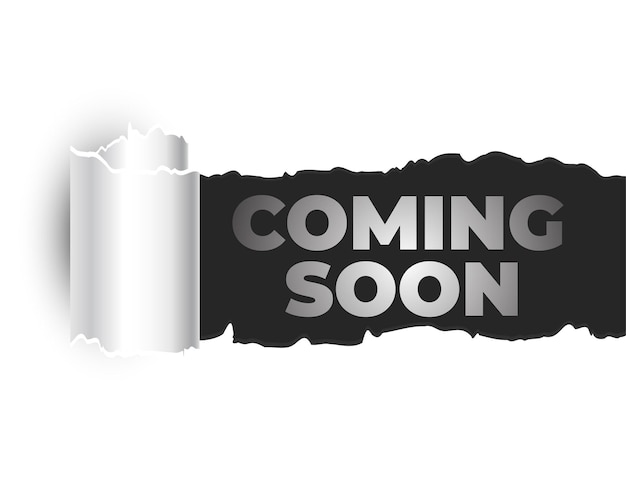
Manual Creation
For more complex scenarios, you can create them manually by writing a JSON file or TypeScript definition:
- Create a new JSON file following the scenario structure
- Define the transaction details and execution steps
- Import the scenario into the EVM Visualizer using the "Import Scenario" option
Best Practices for Scenario Creation
To create high-quality, educational scenarios, follow these best practices:
Ensure Accuracy
Make sure your scenario accurately represents how the EVM would execute the transaction. This includes correct opcode sequences, stack manipulations, and state changes.
Appropriate Granularity
Choose an appropriate level of detail for your steps. Too many steps can be overwhelming, while too few might skip important details. Aim for 5-15 steps for most scenarios.
Clear Descriptions
Write clear, concise descriptions for each step that explain what's happening and why. This helps users understand the purpose of each operation.
Educational Value
Focus on scenarios that demonstrate important concepts or common patterns in Ethereum development. Examples include token transfers, flash loans, or common security vulnerabilities.
Contributing Scenarios
We welcome contributions of high-quality scenarios to the EVM Visualizer project. To contribute a scenario:
- Create your scenario following the guidelines above
- Export it as a JSON file
- Submit a pull request to the EVM Visualizer repository, adding your scenario to the
scenarios/community
directory - Include a brief description of what your scenario demonstrates in the PR description
For more detailed contribution guidelines, see our Contributing Guide.
Example Scenarios
To help you get started, here are some example scenarios that demonstrate different aspects of EVM execution:
Simple ETH Transfer
A basic scenario showing a transfer of ETH from one address to another. This demonstrates the fundamental flow of a transaction through the EVM.
ERC-20 Token Transfer
Shows how an ERC-20 token transfer works, including the function call encoding, storage access, and event emission.
Contract Deployment
Demonstrates how contract creation works in the EVM, including bytecode execution and storage initialization.