EVM Visualizer Developer Guide
Learn how to extend, customize, and contribute to the EVM Visualizer project.
Architecture Overview
The EVM Visualizer is built with a modular architecture that allows for easy extension and customization. The core components are:
- EVMVisualizationDashboard: The main container component that orchestrates the visualization.
- EVMCanvas: The interactive canvas that displays the EVM components and their relationships.
- Custom Nodes: Specialized components for visualizing different aspects of the EVM (Transaction, Stack, Memory, Storage, etc.).
- Simulation Hooks: React hooks that manage the simulation state and logic.
- Detail Panels: Components that display detailed information about each EVM component.
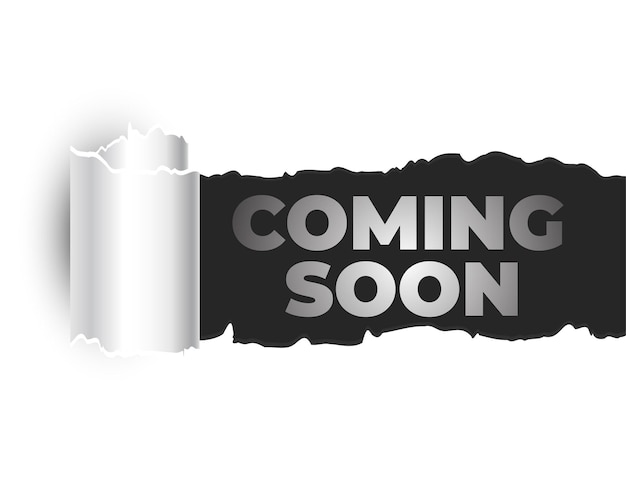
Module System
The EVM Visualizer uses a module system to allow for easy extension and customization. Modules are self-contained components that can be added to the visualization to provide additional functionality.
Module Types
There are several types of modules that can be created:
- Visualization Modules: Add new visual components to the canvas.
- Detail Modules: Add new panels to display detailed information.
- Simulation Modules: Extend the simulation logic with new features.
- Scenario Modules: Add pre-configured transaction scenarios.
Creating Custom Modules
To create a custom module, you need to implement the appropriate interface and register it with the visualizer. Here's an example of creating a custom visualization module:
import { Handle, Position } from "reactflow"
import { Card, CardContent } from "@/components/ui/card"
import { Info } from 'lucide-react'
import type { CustomNodeProps } from "evm-visualizer"
// Define the props for your custom node
interface MyCustomNodeProps {
data: {
customData: any
isActive: boolean
onClick: () => void
}
}
// Create your custom node component
export function MyCustomNode({ data }: MyCustomNodeProps) {
const { customData, isActive, onClick } = data
return (
<>
<Handle type="target" position={Position.Top} className="w-3 h-3 bg-blue-500" />
<Handle type="source" position={Position.Bottom} className="w-3 h-3 bg-blue-500" />
<Card
className={`min-w-[220px] border-blue-200 bg-blue-50 dark:border-blue-900 dark:bg-blue-950/30 shadow-md cursor-pointer transition-all duration-300 ${
isActive ? "ring-2 ring-blue-500 dark:ring-blue-400" : ""
}`}
onClick={onClick}
>
<CardContent className="p-3">
<div className="flex items-center justify-between mb-2">
<div className="text-sm font-semibold text-blue-800 dark:text-blue-300">My Custom Node</div>
<Info className="h-4 w-4 text-blue-500" />
</div>
{/* Your custom node content here */}
<div className="text-sm text-slate-600 dark:text-slate-400">
{customData ? JSON.stringify(customData) : "No data available"}
</div>
</CardContent>
</Card>
</>
)
}
Then, register your custom node with the visualizer:
import { EVMVisualizationDashboard } from 'evm-visualizer';
import { MyCustomNode } from './MyCustomNode';
function App() {
// Define custom node types
const customNodeTypes = {
myCustomNode: MyCustomNode,
};
// Define custom node data
const customNodes = [
{
id: "myCustomNode",
type: "myCustomNode",
data: {
customData: { key: "value" },
isActive: true,
onClick: () => console.log("Custom node clicked"),
},
position: { x: 400, y: 200 },
},
];
return (
<div className="min-h-screen bg-slate-50 dark:bg-slate-900">
<EVMVisualizationDashboard
customNodeTypes={customNodeTypes}
customNodes={customNodes}
/>
</div>
);
}
Creating Custom Scenarios
Scenarios are pre-configured transaction simulations that demonstrate specific aspects of the EVM. You can create custom scenarios to showcase particular features or behaviors.
import { EVMScenario } from 'evm-visualizer';
// Define a custom scenario
export const myCustomScenario: EVMScenario = {
name: "Custom Token Transfer",
description: "Demonstrates a simple ERC-20 token transfer",
transaction: {
from: "0x742d35Cc6634C0532925a3b844Bc454e4438f44e",
to: "0x1234567890123456789012345678901234567890", // Token contract address
value: "0",
gasLimit: 60000,
gasPrice: 20,
data: "0xa9059cbb000000000000000000000000abcdef1234567890abcdef1234567890abcdef00000000000000000000000000000000000000000000000000000000000000064", // transfer(address,uint256)
nonce: 5,
},
steps: [
{
description: "Transaction initiated",
opcodes: ["PUSH1 0x60", "PUSH1 0x40", "MSTORE"],
stack: ["0x60", "0x40"],
memory: "0x0000...0060",
storage: {},
},
{
description: "Decode function selector",
opcodes: ["CALLDATALOAD", "PUSH4 0xffffffff", "AND"],
stack: ["0xa9059cbb"],
memory: "0x0000...0060",
storage: {},
},
// More steps...
],
};
// Use the custom scenario
import { EVMVisualizationDashboard } from 'evm-visualizer';
import { myCustomScenario } from './myCustomScenario';
function App() {
return (
<div className="min-h-screen bg-slate-50 dark:bg-slate-900">
<EVMVisualizationDashboard scenario={myCustomScenario} />
</div>
);
}
Contributing to the Project
We welcome contributions to the EVM Visualizer project! Whether you're fixing bugs, adding features, or improving documentation, your help is appreciated.
Development Setup
To set up the project for development:
# Clone the repository
git clone https://github.com/All-Khwarizmi/evm-visualization
cd evm-visualizer
# Install dependencies
npm install
# Start the development server
npm run dev
Pull Requests
When submitting a pull request, please make sure to follow the project's coding standards and include tests for any new functionality. All pull requests should be made against the main
branch.